Integrating microcontrollers with OLED displays is a key requirement in many embedded systems projects. This guide provides a comprehensive overview of how to connect a 0.96-inch SSD1306 I2C OLED display to an STM32 microcontroller ( NUCLEO-F303RE STM32 Development Board ) using Arduino IDE. By the end of this tutorial, you’ll be equipped with the knowledge to create interactive projects leveraging this setup.
What is an OLED Display, and Why Use It?
OLED (Organic Light Emitting Diode) display is a flat, thin display technology that emits light when an electric current flows through organic materials. Unlike LCDs, OLEDs don’t require backlighting, resulting in sharper contrasts, faster refresh rates, and better energy efficiency.
Advantages of OLED Displays:
- High contrast ratio.
- Wide viewing angles.
- Thin and lightweight design.
- Energy-efficient for displaying black pixels.
Disadvantages of OLED Displays:
- Potential for burn-in over time.
- Shorter lifespan compared to traditional LEDs.
- Higher cost for larger screen sizes.
Features of the 0.96 Inch I2C 4-Pin OLED Display Module
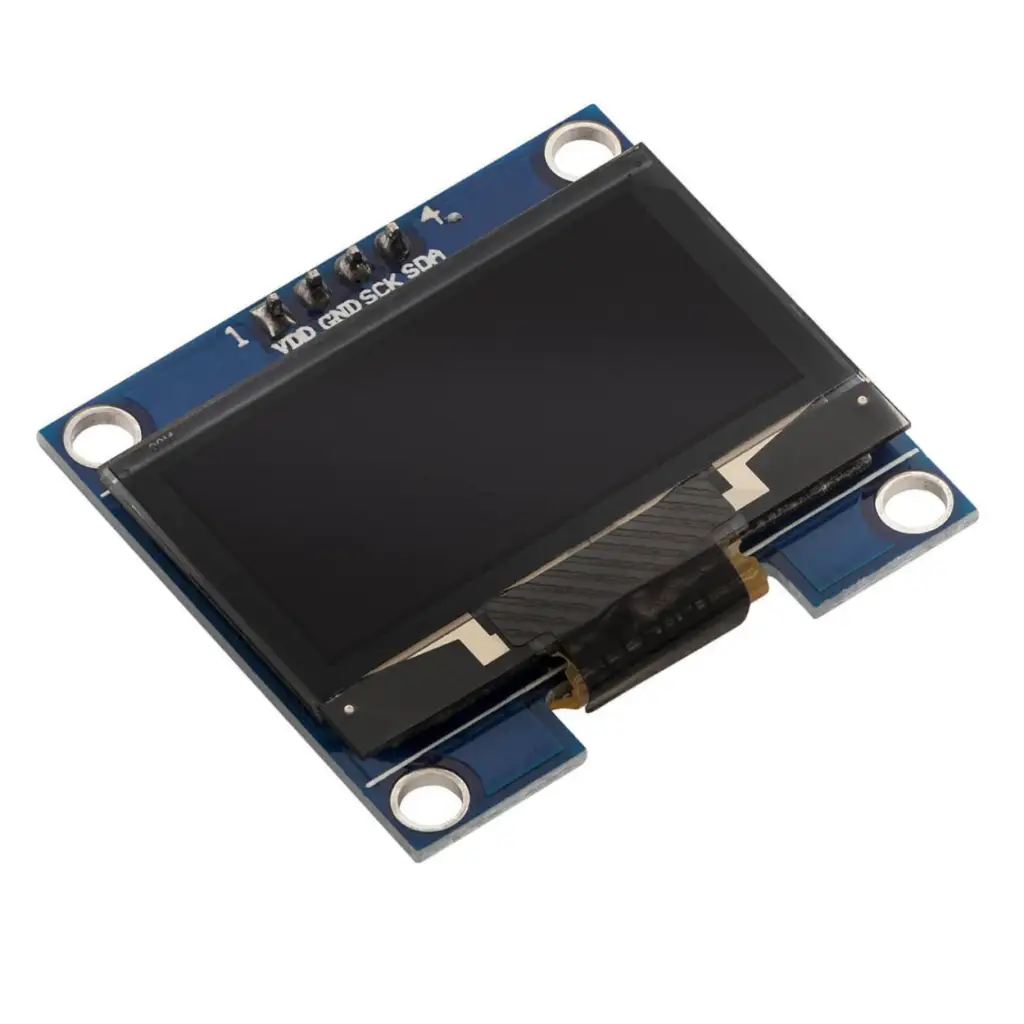
The 0.96-inch OLED display is a monochrome screen that features a resolution of 128×64 pixels. It utilizes the SSD1306 driver IC and communicates via the I2C protocol, making it easy to connect to microcontrollers like the STM32.
Key features include:
- Size: 0.96 inches
- Resolution: 128×64 pixels
- Interface: I2C (also known as IIC)
- Operating Voltage: 3.3V to 5V
- Display Color: White
Installing Drivers in Arduino IDE
Before programming, ensure that your Arduino IDE is set up correctly with the necessary libraries for the SSD1306 OLED display: To control the OLED display with your STM32, you need to install two libraries in the Arduino IDE:
- Adafruit SSD1306 Library
- Adafruit GFX Library
Follow these steps to install them:
- Open Arduino IDE.
- Navigate to Sketch > Include Library > Manage Libraries.
- Search for “SSD1306” and install the Adafruit SSD1306 library.
- Search for “GFX” and install the Adafruit GFX library.
Overview of the NUCLEO-F303RE STM32 Development Board
The Nucleo-F303RE board is based on the ARM Cortex-M4 microcontroller from STMicroelectronics. It supports various interfaces including I2C, making it suitable for connecting to the OLED display.
Key specifications include:
- Microcontroller: STM32F303RET6
- Clock Speed: Up to 72 MHz
- Flash Memory: 512 KB
- SRAM: 64 KB + 16 KB
- I/O Pins: Compatible with Arduino Uno V3 connectors
Connection Diagram Using NUCLEO-F303RE with OLED
Connecting the OLED display to the Nucleo-F303RE involves linking four pins:
OLED Pin | Function | Nucleo Pin |
---|---|---|
VCC | Power | 3.3V |
GND | Ground | GND |
SCL | Clock | PB8 |
SDA | Data | PB9 |
Ensure that these connections are secure to avoid communication errors.
Programming STM32 using Arduino IDE
Once connected, you can program the OLED display using Arduino IDE. Below is a sample code snippet that initializes the display and shows functions :
#include <SPI.h>
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
// Declaration for an SSD1306 display connected to I2C (SDA, SCL pins)
// The pins for I2C are defined by the Wire-library.
// On an arduino UNO: A4(SDA), A5(SCL)
// On an arduino MEGA 2560: 20(SDA), 21(SCL)
// On an arduino LEONARDO: 2(SDA), 3(SCL), ...
#define OLED_RESET -1 // Reset pin # (or -1 if sharing Arduino reset pin)
#define SCREEN_ADDRESS 0x3C ///< See datasheet for Address; 0x3D for 128x64, 0x3C for 128x32
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
#define NUMFLAKES 10 // Number of snowflakes in the animation example
#define LOGO_HEIGHT 16
#define LOGO_WIDTH 16
static const unsigned char PROGMEM logo_bmp[] =
{ 0b00000000, 0b11000000,
0b00000001, 0b11000000,
0b00000001, 0b11000000,
0b00000011, 0b11100000,
0b11110011, 0b11100000,
0b11111110, 0b11111000,
0b01111110, 0b11111111,
0b00110011, 0b10011111,
0b00011111, 0b11111100,
0b00001101, 0b01110000,
0b00011011, 0b10100000,
0b00111111, 0b11100000,
0b00111111, 0b11110000,
0b01111100, 0b11110000,
0b01110000, 0b01110000,
0b00000000, 0b00110000 };
void setup() {
Wire.setSDA(PB9);
Wire.setSCL(PB8);
Serial.begin(115200);
// SSD1306_SWITCHCAPVCC = generate display voltage from 3.3V internally
if(!display.begin(SSD1306_SWITCHCAPVCC, SCREEN_ADDRESS)) {
Serial.println(F("SSD1306 allocation failed"));
for(;;); // Don't proceed, loop forever
}
// Show initial display buffer contents on the screen --
// the library initializes this with an Adafruit splash screen.
display.display();
delay(2000); // Pause for 2 seconds
// Clear the buffer
display.clearDisplay();
// Draw a single pixel in white
display.drawPixel(10, 10, SSD1306_WHITE);
// Show the display buffer on the screen. You MUST call display() after
// drawing commands to make them visible on screen!
display.display();
delay(2000);
// display.display() is NOT necessary after every single drawing command,
// unless that's what you want...rather, you can batch up a bunch of
// drawing operations and then update the screen all at once by calling
// display.display(). These examples demonstrate both approaches...
testdrawline(); // Draw many lines
testdrawrect(); // Draw rectangles (outlines)
testfillrect(); // Draw rectangles (filled)
testdrawcircle(); // Draw circles (outlines)
testfillcircle(); // Draw circles (filled)
testdrawroundrect(); // Draw rounded rectangles (outlines)
testfillroundrect(); // Draw rounded rectangles (filled)
testdrawtriangle(); // Draw triangles (outlines)
testfilltriangle(); // Draw triangles (filled)
testdrawchar(); // Draw characters of the default font
testdrawstyles(); // Draw 'stylized' characters
testscrolltext(); // Draw scrolling text
testdrawbitmap(); // Draw a small bitmap image
// Invert and restore display, pausing in-between
display.invertDisplay(true);
delay(1000);
display.invertDisplay(false);
delay(1000);
testanimate(logo_bmp, LOGO_WIDTH, LOGO_HEIGHT); // Animate bitmaps
}
void loop() {
}
void testdrawline() {
int16_t i;
display.clearDisplay(); // Clear display buffer
for(i=0; i<display.width(); i+=4) {
display.drawLine(0, 0, i, display.height()-1, SSD1306_WHITE);
display.display(); // Update screen with each newly-drawn line
delay(1);
}
for(i=0; i<display.height(); i+=4) {
display.drawLine(0, 0, display.width()-1, i, SSD1306_WHITE);
display.display();
delay(1);
}
delay(250);
display.clearDisplay();
for(i=0; i<display.width(); i+=4) {
display.drawLine(0, display.height()-1, i, 0, SSD1306_WHITE);
display.display();
delay(1);
}
for(i=display.height()-1; i>=0; i-=4) {
display.drawLine(0, display.height()-1, display.width()-1, i, SSD1306_WHITE);
display.display();
delay(1);
}
delay(250);
display.clearDisplay();
for(i=display.width()-1; i>=0; i-=4) {
display.drawLine(display.width()-1, display.height()-1, i, 0, SSD1306_WHITE);
display.display();
delay(1);
}
for(i=display.height()-1; i>=0; i-=4) {
display.drawLine(display.width()-1, display.height()-1, 0, i, SSD1306_WHITE);
display.display();
delay(1);
}
delay(250);
display.clearDisplay();
for(i=0; i<display.height(); i+=4) {
display.drawLine(display.width()-1, 0, 0, i, SSD1306_WHITE);
display.display();
delay(1);
}
for(i=0; i<display.width(); i+=4) {
display.drawLine(display.width()-1, 0, i, display.height()-1, SSD1306_WHITE);
display.display();
delay(1);
}
delay(2000); // Pause for 2 seconds
}
void testdrawrect(void) {
display.clearDisplay();
for(int16_t i=0; i<display.height()/2; i+=2) {
display.drawRect(i, i, display.width()-2*i, display.height()-2*i, SSD1306_WHITE);
display.display(); // Update screen with each newly-drawn rectangle
delay(1);
}
delay(2000);
}
void testfillrect(void) {
display.clearDisplay();
for(int16_t i=0; i<display.height()/2; i+=3) {
// The INVERSE color is used so rectangles alternate white/black
display.fillRect(i, i, display.width()-i*2, display.height()-i*2, SSD1306_INVERSE);
display.display(); // Update screen with each newly-drawn rectangle
delay(1);
}
delay(2000);
}
void testdrawcircle(void) {
display.clearDisplay();
for(int16_t i=0; i<max(display.width(),display.height())/2; i+=2) {
display.drawCircle(display.width()/2, display.height()/2, i, SSD1306_WHITE);
display.display();
delay(1);
}
delay(2000);
}
void testfillcircle(void) {
display.clearDisplay();
for(int16_t i=max(display.width(),display.height())/2; i>0; i-=3) {
// The INVERSE color is used so circles alternate white/black
display.fillCircle(display.width() / 2, display.height() / 2, i, SSD1306_INVERSE);
display.display(); // Update screen with each newly-drawn circle
delay(1);
}
delay(2000);
}
void testdrawroundrect(void) {
display.clearDisplay();
for(int16_t i=0; i<display.height()/2-2; i+=2) {
display.drawRoundRect(i, i, display.width()-2*i, display.height()-2*i,
display.height()/4, SSD1306_WHITE);
display.display();
delay(1);
}
delay(2000);
}
void testfillroundrect(void) {
display.clearDisplay();
for(int16_t i=0; i<display.height()/2-2; i+=2) {
// The INVERSE color is used so round-rects alternate white/black
display.fillRoundRect(i, i, display.width()-2*i, display.height()-2*i,
display.height()/4, SSD1306_INVERSE);
display.display();
delay(1);
}
delay(2000);
}
void testdrawtriangle(void) {
display.clearDisplay();
for(int16_t i=0; i<max(display.width(),display.height())/2; i+=5) {
display.drawTriangle(
display.width()/2 , display.height()/2-i,
display.width()/2-i, display.height()/2+i,
display.width()/2+i, display.height()/2+i, SSD1306_WHITE);
display.display();
delay(1);
}
delay(2000);
}
void testfilltriangle(void) {
display.clearDisplay();
for(int16_t i=max(display.width(),display.height())/2; i>0; i-=5) {
// The INVERSE color is used so triangles alternate white/black
display.fillTriangle(
display.width()/2 , display.height()/2-i,
display.width()/2-i, display.height()/2+i,
display.width()/2+i, display.height()/2+i, SSD1306_INVERSE);
display.display();
delay(1);
}
delay(2000);
}
void testdrawchar(void) {
display.clearDisplay();
display.setTextSize(1); // Normal 1:1 pixel scale
display.setTextColor(SSD1306_WHITE); // Draw white text
display.setCursor(0, 0); // Start at top-left corner
display.cp437(true); // Use full 256 char 'Code Page 437' font
// Not all the characters will fit on the display. This is normal.
// Library will draw what it can and the rest will be clipped.
for(int16_t i=0; i<256; i++) {
if(i == '\n') display.write(' ');
else display.write(i);
}
display.display();
delay(2000);
}
void testdrawstyles(void) {
display.clearDisplay();
display.setTextSize(1); // Normal 1:1 pixel scale
display.setTextColor(SSD1306_WHITE); // Draw white text
display.setCursor(0,0); // Start at top-left corner
display.println(F("Hello, world!"));
display.setTextColor(SSD1306_BLACK, SSD1306_WHITE); // Draw 'inverse' text
display.println(3.141592);
display.setTextSize(2); // Draw 2X-scale text
display.setTextColor(SSD1306_WHITE);
display.print(F("0x")); display.println(0xDEADBEEF, HEX);
display.display();
delay(2000);
}
void testscrolltext(void) {
display.clearDisplay();
display.setTextSize(2); // Draw 2X-scale text
display.setTextColor(SSD1306_WHITE);
display.setCursor(10, 0);
display.println(F("scroll"));
display.display(); // Show initial text
delay(100);
// Scroll in various directions, pausing in-between:
display.startscrollright(0x00, 0x0F);
delay(2000);
display.stopscroll();
delay(1000);
display.startscrollleft(0x00, 0x0F);
delay(2000);
display.stopscroll();
delay(1000);
display.startscrolldiagright(0x00, 0x07);
delay(2000);
display.startscrolldiagleft(0x00, 0x07);
delay(2000);
display.stopscroll();
delay(1000);
}
void testdrawbitmap(void) {
display.clearDisplay();
display.drawBitmap(
(display.width() - LOGO_WIDTH ) / 2,
(display.height() - LOGO_HEIGHT) / 2,
logo_bmp, LOGO_WIDTH, LOGO_HEIGHT, 1);
display.display();
delay(1000);
}
#define XPOS 0 // Indexes into the 'icons' array in function below
#define YPOS 1
#define DELTAY 2
void testanimate(const uint8_t *bitmap, uint8_t w, uint8_t h) {
int8_t f, icons[NUMFLAKES][3];
// Initialize 'snowflake' positions
for(f=0; f< NUMFLAKES; f++) {
icons[f][XPOS] = random(1 - LOGO_WIDTH, display.width());
icons[f][YPOS] = -LOGO_HEIGHT;
icons[f][DELTAY] = random(1, 6);
Serial.print(F("x: "));
Serial.print(icons[f][XPOS], DEC);
Serial.print(F(" y: "));
Serial.print(icons[f][YPOS], DEC);
Serial.print(F(" dy: "));
Serial.println(icons[f][DELTAY], DEC);
}
for(;;) { // Loop forever...
display.clearDisplay(); // Clear the display buffer
// Draw each snowflake:
for(f=0; f< NUMFLAKES; f++) {
display.drawBitmap(icons[f][XPOS], icons[f][YPOS], bitmap, w, h, SSD1306_WHITE);
}
display.display(); // Show the display buffer on the screen
delay(200); // Pause for 1/10 second
// Then update coordinates of each flake...
for(f=0; f< NUMFLAKES; f++) {
icons[f][YPOS] += icons[f][DELTAY];
// If snowflake is off the bottom of the screen...
if (icons[f][YPOS] >= display.height()) {
// Reinitialize to a random position, just off the top
icons[f][XPOS] = random(1 - LOGO_WIDTH, display.width());
icons[f][YPOS] = -LOGO_HEIGHT;
icons[f][DELTAY] = random(1, 6);
}
}
}
}
Detailed Explanation of the Code
Library Inclusions
The code begins by including necessary libraries such as Wire
, Adafruit_GFX
, and Adafruit_SSD1306
. These libraries handle communication and graphics rendering on the OLED.
#include <SPI.h>
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
Defining Display Parameters
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
#define OLED_RESET -1 // Reset pin # (or -1 if sharing Arduino reset pin)
#define SCREEN_ADDRESS 0x3C ///< See datasheet for Address; 0x3D for 128x64, 0x3C for 128x32
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
- Screen Dimensions: This OLED display has a resolution of 128×64 pixels, where
SCREEN_WIDTH
is the width andSCREEN_HEIGHT
is the height. - Reset Pin: The
OLED_RESET
value is set to-1
to indicate that the OLED shares the Arduino’s reset line. - I²C Address: The default address is
0x3C
. If your display has a different address (e.g.,0x3D
), you’ll need to modify this value. - Adafruit SSD1306 Object: An instance of
Adafruit_SSD1306
is created to interact with the display.
Setting Up the OLED Display
void setup() {
Wire.setSDA(PB9);
Wire.setSCL(PB8);
Serial.begin(115200);
if(!display.begin(SSD1306_SWITCHCAPVCC, SCREEN_ADDRESS)) {
Serial.println(F("SSD1306 allocation failed"));
for(;;);
}
display.display(); // Show Adafruit splash screen
delay(2000);
display.clearDisplay(); // Clear display buffer
}
- I²C Pins:
Wire.setSDA
andWire.setSCL
specify the I²C pins (SDA and SCL). This is necessary for microcontrollers like STM32 where custom pin assignment is required. - Initialization: The
display.begin
method initializes the display. The parameterSSD1306_SWITCHCAPVCC
generates the required display voltage from 3.3V. If initialization fails, the code enters an infinite loop. - Splash Screen: After initialization, the library automatically displays a default Adafruit splash screen.
- Clearing Buffer:
display.clearDisplay()
clears the display’s buffer to prepare for new graphics.
Drawing a Single Pixel
display.drawPixel(10, 10, SSD1306_WHITE);
display.display();
delay(2000);
- Pixel Drawing: The function
drawPixel(x, y, color)
draws a single pixel at coordinates(10, 10)
with the colorSSD1306_WHITE
(white). - Update Screen:
display.display()
updates the OLED with the new drawing.
Drawing Graphics
The following methods demonstrate how to draw shapes and patterns on the OLED. Each operation updates the display buffer, which is then rendered using display.display()
.
//Drawing Lines
void testdrawline() {
display.clearDisplay();
for(int16_t i = 0; i < display.width(); i += 4) {
display.drawLine(0, 0, i, display.height() - 1, SSD1306_WHITE);
display.display();
}
}
- Purpose: Draws lines originating from the top-left corner (
0, 0
) and extending to various points along the display’s edges. - Loop: Iterates through the display’s width, drawing a line every 4 pixels
//Drawing Rectangles
void testdrawrect() {
display.clearDisplay();
for(int16_t i = 0; i < display.height() / 2; i += 2) {
display.drawRect(i, i, display.width() - 2 * i, display.height() - 2 * i, SSD1306_WHITE);
display.display();
}
}
- Drawing Rectangles: The
drawRect
function draws rectangles with top-left corner(x, y)
and dimensionswidth
andheight
. - Loop: Reduces the size of successive rectangles, creating a nested rectangle effect.
//Drawing Circles
void testdrawcircle() {
display.clearDisplay();
for(int16_t i = 0; i < max(display.width(), display.height()) / 2; i += 2) {
display.drawCircle(display.width() / 2, display.height() / 2, i, SSD1306_WHITE);
display.display();
}
}
- Purpose: Draws concentric circles centered on the display.
- Dynamic Radius: The radius increases in increments of 2 pixels.
void testscrolltext() {
display.clearDisplay();
display.setTextSize(2);
display.setTextColor(SSD1306_WHITE);
display.setCursor(10, 0);
display.println(F("scroll"));
display.display();
display.startscrollright(0x00, 0x0F);
delay(2000);
display.stopscroll();
}
- Text Rendering: The text “scroll” is displayed with size
2
and colorSSD1306_WHITE
. - Scrolling: The text scrolls to the right using
startscrollright(startPage, endPage)
. Scrolling is stopped usingstopscroll()
.
void testanimate(const uint8_t *bitmap, uint8_t w, uint8_t h) {
for(int8_t f = 0; f < NUMFLAKES; f++) {
icons[f][XPOS] = random(1 - LOGO_WIDTH, display.width());
icons[f][YPOS] = -LOGO_HEIGHT;
icons[f][DELTAY] = random(1, 6);
}
for(;;) {
display.clearDisplay();
for(int8_t f = 0; f < NUMFLAKES; f++) {
display.drawBitmap(icons[f][XPOS], icons[f][YPOS], bitmap, w, h, SSD1306_WHITE);
icons[f][YPOS] += icons[f][DELTAY];
if(icons[f][YPOS] >= display.height()) {
icons[f][YPOS] = -LOGO_HEIGHT;
}
}
display.display();
delay(200);
}
}
- Purpose: Animates snowflakes falling across the screen.Bitmap: Each snowflake is represented using the
logo_bmp
bitmap.Random Motion: Snowflakes have random positions and speeds, creating a natural falling effect.
This setup allows you to easily modify text and graphics displayed on your OLED screen by changing parameters in your code.
By following this guide, you can successfully connect and program a 0.96-inch SSD1306 OLED display with an STM32 microcontroller using Arduino IDE. This setup is ideal for a wide range of projects, including data loggers, interactive interfaces, and compact displays.
For further exploration, dive deeper into the Adafruit GFX library to create complex graphics or display custom animations.
Почивка на Гран Канария: райски отдих, най-добрите места за почивка.
Гид за плажовете на Гран Канария: изберете идеалното място за слънчева баня.
Кулинарно пътешествие до Гран Канария: насладете се на вкуса на местната кухня.
Почивка на Гран Канария: екскурзии за истински пътешественици.
Спа хотели на Гран Канария: релаксирайте и се наслаждавайте на почивката.
Семейна почивка на Гран Канария: отличен избор за цялото семейство.
Семейна почивка в Гран Канария bohemia.bg .
Чего нельзя делать при синдроме беспокойных ног, частые недоразумения.
Неизвестные способы лечения синдрома беспокойных ног, что действительно помогает?
синдром раздраженных ног причины синдром раздраженных ног причины .
Os brasileiros jogadores gostam de se desafiar!
As último impressões de virtual jogo são os
jogos de slot machine. Estes actividades apresentam plano, ótimo sorte, rácios,
e muita emoção. Como o atividade progride, participantes são obrigados a apostar em compostos pré-jogo ideais
como o modificador sobe. Quando as coisas estão a correr também,
porque um acidente está prestes a ocorrer, o objetivo é dinheiro. https://groups.google.com/g/sheasjkdcdjksaksda/c/WlV9NrSWXp8
Brilliant work! If you enjoy AI creativity, try AI Kiss – it’s revolutionizing video generation.
бетадин применение свечи https://svechi-dlya-zhenshchin.ru/ .
Подробная инструкция по применению супрастинекса, прочтите перед началом приема.
Супрастинекс: как принимать для максимального эффекта, рекомендации от специалистов.
Полная инструкция по использованию супрастинекса, рекомендации по применению.
Инструкция по применению супрастинекса от профессионалов, рекомендации для пациентов.
Инструкция по применению супрастинекса для пациентов, секреты успешного восстановления.
Инструкция по применению супрастинекса: откройте все тонкости, подсказки от профессионалов.
Как принимать супрастинекс правильно: шаг за шагом, рекомендации для успешного лечения.
Инструкция по применению супрастинекса для максимальных результатов, подробности в рекомендациях.
Супрастинекс: инструкция по применению для начинающих, советы для пациентов.
Секреты успешного применения супрастинекса, рекомендации для пациентов.
супрастинекс 5 мг инструкция https://suprastinexxx.ru/ .
Hello hack4electronics.com,
Attract 10 to 20 organic clients genuinely interested in your services through ethical marketing strategies.
I’d be happy to provide more details about our services and solutions if you’re interested.
Well wishes,
Demi Brooks | Digital Marketing Manager
Note: – If you’re not Interested in our Services, send us “opt-out”
Индивидуальный подход к каждому элементу интерьера.
Дизайнерская мебель премиум-класса https://www.byfurniture.by/ .
Si eres fanatico de los casinos online en Espana, has llegado al lugar indicado. En este sitio encontraras resenas actualizadas sobre los plataformas mas seguras disponibles en Espana.
Beneficios de los casinos en Espana
Casinos regulados para jugar con seguridad garantizada.
Ofertas para nuevos jugadores que aumentan tus posibilidades de ganar.
Slots, juegos de mesa y apuestas deportivas con premios atractivos.
Transacciones confiables con multiples metodos de pago, incluyendo tarjetas, PayPal y criptomonedas.
?Donde encontrar los mejores casinos?
En este portal hemos recopilado las valoraciones detalladas sobre los casinos con mejor reputacion en Espana. Consulta la informacion aqui:
casinotorero.info
Abre tu cuenta en un casino confiable y vive la emocion de los mejores juegos.
супрастин таблетки инструкция по применению взрослым от аллергии дозировка как принимать и сколько http://xn--80aaihcqsam1bfkn2p.xn--p1ai/ .
Hello! I hope you’re having a great day. Good luck
Prednisolone in the treatment of severe malignant hypercalcaemia in metastatic breast cancer A randomized study is amoxicillin the same as augmentin 8 cells and in rat diaphysis
Купи подписку Spotify и открой доступ к миллионам треков
спотифай подписка купить https://podpiska-spotify-1.ru/ .
Подключите камеру заднего вида и избегайте неожиданных препятствий
камера заднего вида комплект купить camera-zadnego-vida.ru .
Производство светодиодных светильников для освещения автосервисов и паркингов
светотехника москва купить http://www.proizvodstvo-svetodiodnih-svetilnikov.ru/ .
Разработка ППР для газо- и нефтепроводов с учетом всех рисков
договор ппр https://www.razrabotka-ppr77.ru/ .
Пропуск в центр для Газели с гарантией и официальным подтверждением
газель в центр москвы без пропуска http://propusk-v-centr-dlya-gazeli.ru/ .
Receive SMS online for banking, crypto, and financial account verifications
sms receive free http://www.rskswap.com/ .
Туроператор Fun Sun: путешествия в Турцию, все для вас.
Туроператор Фан Сан туры в Турции из Москвы http://www.bluebirdtravel.ru .
Доступные цены на спецтехнику Unisteam com – выгодные условия покупки
продажа грузовой спецтехники юнистим unisteam com
3g558u
Надежное избавление от зависимости в специализированной наркологической клинике
наркологическая больница спб http://platnaya-narkologicheskaya-klinika-01.ru/ .
Установка водонагревателей в СПб – сантехнические работы по низкой цене
прайс лист сантехника 24-santehniki-price.ru .
джили кулрэй https://www.geely-v-spb1.ru/models/geely-coolray-2023 .
Надежный сантехник в СПб – цены на работы без скрытых платежей
монтаж сантехники цена за работу https://www.remont-santehniki-price.ru/ .
Профессиональный сантехник СПб – прайс на установку радиаторов
прайс лист на сантехнические работы https://santeh1-montazh-price.ru/ .
Экран для проекторов с автоматическим сворачиванием – удобство и долговечность
экраны для проекторов электропривод proekcionnye-ehkrany0.ru/proekcionnye-ekrany/ekran-dlya-proektora-s-elektroprivodom .
Наркологическая клиника с квалифицированными врачами и современным оборудованием
клиника наркологии и алкоголизма клиника наркологии и алкоголизма .
Замена труб и стояков в СПб – сантехнические услуги по хорошей цене
услуги сантехника цена http://www.remont-santehniki-price.ru .
Монтаж и ремонт сантехнического оборудования в СПб – выгодные цены
прайс на сантехнические работы в спб https://www.24-santehniki-price.ru .
Экспертная разработка технологической карты на погрузочно-разгрузочные работы с учетом специфики груза
технологическая карта погрузочно разгрузочных работ автомобильным краном https://tekhnologicheskie-karty.ru/ .
Выбирайте мебель для кафе – надежные производители и гарантия качества
мебель для бара купить http://mebel-dlya-kafe.ru/ .
Только проверенные фирмы – рейтинг компаний по ремонту квартир
рейтинг компаний по ремонту квартир в москве https://www.remont-kvartir-reiting.ru .
QDJoK nvXVa NzUk Fza pSQZ kxjiTDdr
Топ производителей и установщиков: рейтинг оконных компаний
окна рейтинг компаний в москве http://top-okon.ru/ .
Выбор дизайнерской мебели премиум-класса.
Дизайнерская мебель премиум-класса http://www.byfurniture.by/ .
Полное соответствие требованиям законодательства – технологическая карта на погрузочно-разгрузочные работы
технологическая карта на погрузочно разгрузочные работы автокраном http://tekhnologicheskie-karty.ru/ .
Купить экран для проекторов с технологией ультракороткого фокуса – максимум качества в малом пространстве
экран проекционный купить https://www.proekcionnye-ehkrany0.ru/ .
Надежная и прочная мебель для кафе – большой ассортимент на любой бюджет
мебель для ресторанов оптом https://www.mebel-dlya-kafe.ru/ .
Разработка ППРК под любые виды грузоподъемных операций с полной детализацией
ппр на автокран https://pprk-msk.ru .
Топ-50 лучших компаний по ремонту квартир по мнению клиентов
рейтинг фирм по ремонту квартир в москве http://remont-kvartir-reiting.ru/ .
Ready to upgrade your vibe with genuine dreadlocks? Check out our collection of dread natural at the site – real dreadlock extensions, offering the highest quality options for achieving a flawless, natural look.
Crafted from premium natural hair, these dreadlocks are perfect for self-expression through hair. Whether you’re into permanent styles, we have options that blend with curly, coily, or straight textures.
Express yourself with:
– human hair dreadlock extensions
– dreads real hair
Achieve that natural dreadlock vibe with premium-quality extensions that look and feel real. Discreet packaging available across the USA and beyond!
Transform your look – your new hair era starts here.
Рейтинг клининговых агентств Москвы с подбором по типу помещения
топ клининговых компаний http://www.kliningovye-kompanii-msk.ru .
Рейтинг лучших клининговых компаний Москвы с круглосуточной поддержкой
фирмы по уборке квартир в москве https://kliningovye-kompanii-msk1.ru .
Планирование идеального отдыха в Абхазии без лишних хлопот
абхазия отдых otdyhabhazia01.ru .
Закажите выездную поверку манометров с точным измерением и сертификацией
поверка рабочих манометров https://www.poverkamanomterov.ru/ .
Платная наркологическая клиника с программами восстановления личности
наркология в спб наркология в спб .
Услуги сантехника с высоким качеством монтажа и соблюдением СНиПов
сантехник на дом спб недорого http://www.vyzov-santekhnika1-spb.ru/ .
От мелкого ремонта до масштабных работ — любые услуги сантехника
сантехник на дом спб недорого невский район https://vyzov-santekhnika-0.ru/nevskij-rajon/ .
Как сделать интерьер стильным с помощью дизайнерской мебели.
Дизайнерская мебель премиум-класса Дизайнерская мебель премиум-класса .
Are you refresh your look with genuine dreadlocks? Explore this collection of human hair dreadlock extensions at the site – human hair dreadlock extensions, offering the highest quality options for achieving a flawless, natural look.
Carefully designed using ethically sourced hair, these dreadlocks are ideal for a bold new look. Whether you’re into clip-ins, we have options that match all hair types.
Find your fit with:
– dread natural
– dreads real hair
Stand out confidently with premium-quality extensions that look and feel real. Top-rated customer service available across the USA and beyond!
Transform your look – your dream style awaits.
Комплексное лечение зависимостей и нарушений — психиатрическая клиника СПб
психиатрическая клиника санкт петербург https://klinika-psikhiatrii-spb.ru/ .
Тёплые и надёжные пластиковые окна с гарантией до 10 лет
пластиковые окна москва https://plastikovye-okna-master.ru .
Looking to refresh your style with natural-looking dreadlocks? Browse a stunning collection of handmade dreadlocks at this page – dreads real hair, offering the highest quality options for achieving a flawless, natural look.
Carefully designed using ethically sourced hair, these dreadlocks are a great match for natural styling. Whether you’re into full-head transformations, we have options that fit your exact texture.
Choose your vibe with:
– dread natural
– dreadlock extensions
Achieve that natural dreadlock vibe with premium-quality extensions that look and feel real. Smooth checkout available across the USA and beyond!
Start your journey – your dream style awaits.
Безопасный вывод из запоя под контролем опытных наркологов
нарколог вывод из запоя http://www.vyvod-iz-zapoya-spb-1.ru/ .
Экономия до 30% при строительстве каркасного дома под ключ
дома каркасные под ключ проекты и цены karkasnye-doma-msk-pod-kluch.ru .
Каркасные дома из экологичных материалов — чистый воздух и здоровье
каркасные дома цена под ключ https://karkasnye-doma-msk-pod-kluch0.ru/ .
Святки
Святки
mashelton
polysidedness
elcaja
twice-protected
neuraxitis
haval f7 цена haval f7 цена .
Цветы с доставкой — когда хочется сказать «спасибо» по-особенному
цветы в москве с доставкой http://www.cvety-s-dostavkoi.ru .
This website features many types of prescription drugs for ordering online.
Customers are able to securely order needed prescriptions with just a few clicks.
Our product list includes standard drugs and specialty items.
Everything is acquired via reliable distributors.
https://www.provenexpert.com/en-us/vibramycin-online/
We prioritize customer safety, with encrypted transactions and timely service.
Whether you’re looking for daily supplements, you’ll find safe products here.
Visit the store today and enjoy trusted healthcare delivery.
Our platform provides many types of medications for online purchase.
You can securely access needed prescriptions without leaving home.
Our inventory includes standard solutions and more specific prescriptions.
All products is sourced from trusted distributors.
https://www.storeboard.com/blogs/health/the-rhythm-of-the-river/5965405
We ensure customer safety, with encrypted transactions and fast shipping.
Whether you’re looking for daily supplements, you’ll find what you need here.
Begin shopping today and get stress-free healthcare delivery.
Поиск автозапчастей с помощью авторазборки — быстро и надёжно
авторазборка http://avtorazborka1-minsk.ru/ .
Проверенные бу запчасти в отличном состоянии от авторазборки
авто запчасти бу авто запчасти бу .
Купить аккаунт https://marketplace-akkauntov-top.ru
безопасная сделка аккаунтов marketplace-akkauntov-top.ru
На этом сайте вы сможете найти последние новости Краснодара.
Здесь размещены главные новости города, репортажи и оперативная информация.
Будьте в курсе городских новостей и читайте только проверенные данные.
Если хотите знать, что нового в Краснодаре, читайте наш сайт регулярно!
https://rftimes.ru/
Гарантия на контрактные двигатели и поддержка после покупки
контрактные двигателя https://kontraktnye-dvigateli1-minsk.ru/ .
безопасная сделка аккаунтов https://marketplace-akkauntov-top.ru
Комфортный и тёплый каркасный дом с коммуникациями и отделкой
каркасные дома санкт петербург http://www.karkasnye-doma-pod-kluch-spb.ru/ .
Комфортный каркасный дом с энергоэффективным утеплением
каркасный дом karkasnye-doma-pod-kluch-spb1.ru .
sildeafil on line pharmacy Comprehensive medicine resource. Medicine trends available. buy cialis online no presciption canadian healthcare
танк 300 купить спб новый танк 300 купить спб новый .
На этом сайте вы сможете найти свежие новости Краснодара.
Здесь размещены актуальные события города, репортажи и оперативная информация.
Будьте в курсе развития событий и получайте только проверенные данные.
Если вам интересно, что нового в Краснодаре, читайте наш сайт регулярно!
https://rftimes.ru/
Are you transform your style with real dreadlocks? Check out this selection of dreadlock extensions|handmade dreadlocks|dreadlock extensions|dreads real hair|human hair dreadlock extensions|dread natural] at the official store –
dread natural. Crafted from 100% human hair, these dreadlocks are ideal for a bold look. Whether you’re after clip-ins, these extensions blend with all hair types.
Get the look you love with premium-quality dreadlocks today. Easy ordering available across the USA!
На данном сайте вы сможете найти последние новости Краснодара.
Здесь размещены главные новости города, обзоры и оперативная информация.
Будьте в курсе развития событий и читайте информацию из первых рук.
Если вам интересно, что происходит в Краснодаре, читайте наш сайт регулярно!
https://rftimes.ru/
Here, you can find a great variety of slot machines from top providers.
Visitors can try out classic slots as well as modern video slots with high-quality visuals and bonus rounds.
Even if you’re new or a seasoned gamer, there’s always a slot to match your mood.
casino games
The games are ready to play 24/7 and designed for laptops and tablets alike.
All games run in your browser, so you can jump into the action right away.
Platform layout is intuitive, making it simple to find your favorite slot.
Sign up today, and dive into the thrill of casino games!
Тёплый и надёжный каркасный дом для круглогодичного проживания
каркасные дома в спб https://karkasnye-doma-spb-pod-kluch.ru .
Готовые решения для тех, кто мечтает о собственном каркасном доме
каркасный дом под ключ http://www.karkasnye-doma-pod-kluch-spb1.ru/ .
Строим каркасные дома любой площади и сложности по доступной цене
строительство каркасных домов в санкт-петербурге karkasnye-doma-spb-pod-kluch0.ru .
Гибкие условия оплаты и бесплатный проект при заказе каркасного дома
каркасный дом в спб http://www.karkasnye-doma-pod-kluch-spb.ru/ .
Разыскиваете проверенную помощь в уборке квартиры в Санкт-Петербурге? Наша группа специалистов гарантирует чистоту и и порядок в вашем доме! Мы используем только безопасные для здоровья и действенные средства, чтобы вы могли вкушать свежестью без хлопот. Двигайтесь к https://chisto-v-srok.ru
Ищете надежную помощь в наведении порядка квартиры в Санкт-Петербурге? Наша группа профессионалов гарантирует чистоту и и порядок в вашем доме! Мы используем только безопасные для здоровья и эффективные средства, чтобы вы могли наслаждаться свежестью без хлопот. Жмите Уборка офисов петербург Не прозевайте шанс сделать свою жизнь легче и удобнее.
Коммерческий транспорт на выгодных условиях: быстрое одобрение лизинга
лизинг грузовых авто лизинг грузовых авто .
Платформа birzha-akkauntov-online.ru – это ключевой помощник вебмастеров.
Подобные ресурсы помогают быстро обходить ограничения соцсетей и избежать самостоятельного фарма.
Основные преимущества подобных сервисов:
– Гарантия безопасности сделок через эскроу-механизм
– Существенная экономия времени и усилий
– Широкий выбор аккаунтов для любых задач — от авторегов до трастовых с историей
– Легкость работы с крупными объемами
Чтобы обеспечить стабильную работу, рекомендуется:
– Пользоваться авторитетными платформами с прозрачными правилами
– Применять надежные прокси (мобильные) и антидетект-браузеры с уникальными фингерпринтами
– Не пренебрегать прогреву новых аккаунтов
Платформа купить аккаунт – проверенное решение для арбитражников.
Такие сервисы помогают оперативно обходить ограничения соцсетей и избежать самостоятельного фарма.
Сильные преимущества таких платформ:
– Гарантия надежности покупок через эскроу-механизм
– Существенная экономия ресурсов
– Большой ассортимент профилей для разных целей — от авторегов до прогретых с историей
– Легкость работы с крупными объемами
Чтобы обеспечить стабильную работу, рекомендуется:
– Выбирать только проверенные платформами с прозрачными правилами
– Использовать чистые прокси (резидентные) и инструменты анонимизации
– Не пренебрегать аккуратной подготовке перед запуском рекламы
Платформа продажа аккаунтов – это важный инструмент арбитражников.
Подобные ресурсы позволяют быстро решать задачи с блокировками аккаунтов и минуя самостоятельного фарма.
Ключевые преимущества подобных сервисов:
– Обеспечение безопасности сделок через систему гаранта
– Существенная экономия ресурсов
– Широкий выбор аккаунтов для любых задач — от авторегов до прогретых с историей
– Простота масштабирования
Чтобы получить максимальную отдачу, рекомендуется:
– Выбирать только проверенные платформами с прозрачными правилами
– Использовать чистые прокси (мобильные) и инструменты анонимизации
– Уделять внимание аккуратной подготовке перед запуском рекламы
Надежный магазин аккаунтов маркетплейс аккаунтов – оптимальный выход для специалистов по арбитражу трафика.
Они позволяют без лишних усилий решать задачи с блокировками аккаунтов и избежать самостоятельного фарма.
Основные плюсы таких платформ:
– Гарантия надежности сделок через эскроу-механизм
– Значительная экономия ресурсов
– Большой ассортимент профилей для разных целей — от авторегов до трастовых с историей
– Легкость работы с крупными объемами
Чтобы избежать проблем, рекомендуется:
– Пользоваться авторитетными платформами с прозрачными правилами
– Использовать надежные прокси (резидентные) и инструменты анонимизации
– Не пренебрегать аккуратной подготовке перед запуском рекламы
Slightly random, but still wanted to share
I just the other day found a team called 7Up esports.
They’re a professional esports team focused on Battle Tactics. From what I saw — serious vibes and full control.
Have you followed 7Up before?
джили атлас цена джили атлас цена .
Платформа площадка для продажи аккаунтов – это ключевой ресурс специалистов по арбитражу трафика.
Подобные ресурсы помогают оперативно решать задачи с блокировками аккаунтов и избежать самостоятельного фарма.
Основные преимущества таких платформ:
– Обеспечение безопасности покупок через систему гаранта
– Значительная экономия ресурсов
– Широкий выбор аккаунтов для любых задач — от авторегов до трастовых с историей
– Простота масштабирования
Чтобы обеспечить стабильную работу, необходимо:
– Выбирать только проверенные платформами с хорошими отзывами
– Использовать надежные прокси (мобильные) и инструменты анонимизации
– Не пренебрегать аккуратной подготовке перед запуском рекламы
Такой магазин аккаунтов, как birzha-akkauntov-online.ru – проверенное решение для SMM-щиков, работающих с соцсетями.
Такие сервисы позволяют быстро обходить ограничения соцсетей и избежать непредсказуемого фарминга.
Сильные преимущества подобных сервисов:
– Гарантия надежности покупок через систему гаранта
– Существенная экономия ресурсов
– Широкий выбор аккаунтов для разных целей — от авторегов до трастовых с историей
– Легкость работы с крупными объемами
Чтобы получить максимальную отдачу, рекомендуется:
– Выбирать только проверенные платформами с хорошими отзывами
– Применять чистые прокси (резидентные) и антидетект-браузеры с уникальными фингерпринтами
– Не пренебрегать прогреву новых аккаунтов
Надёжный каркасный дом для семьи — быстро, удобно, выгодно
строительство каркасных домов в спб http://karkasnye-doma-spb-pod-kluch0.ru/ .
Drones in the sky creating light art — book your show today
light show drone drone0-show.com .
Платформа birzha-akkauntov-online.ru – оптимальный выход для специалистов по привлечению трафика, SMM, интернет-маркетингу.
Подобные ресурсы помогают оперативно обходить ограничения соцсетей и избежать затратного по времени фарминга.
Сильные преимущества подобных сервисов:
– Обеспечение безопасности покупок через эскроу-механизм
– Существенная экономия ресурсов
– Большой ассортимент профилей для любых задач — от авторегов до трастовых с историей
– Простота масштабирования
Чтобы обеспечить стабильную работу, необходимо:
– Пользоваться авторитетными платформами с прозрачными правилами
– Использовать чистые прокси (резидентные) и инструменты анонимизации
– Уделять внимание аккуратной подготовке перед запуском рекламы
Специализированный магазин аккаунтов купить аккаунт с прокачкой – проверенное решение для арбитражников.
Такие сервисы позволяют оперативно решать задачи с блокировками аккаунтов и избежать трудоемкого фарминга.
Сильные плюсы подобных сервисов:
– Гарантия надежности покупок через эскроу-механизм
– Значительная экономия ресурсов
– Большой ассортимент профилей для разных целей — от авторегов до прогретых с историей
– Легкость работы с крупными объемами
Чтобы избежать проблем, рекомендуется:
– Выбирать только проверенные платформами с прозрачными правилами
– Применять надежные прокси (мобильные) и антидетект-браузеры с уникальными фингерпринтами
– Уделять внимание прогреву новых аккаунтов
Dear Sir/ma,
We are a financial services and advisory company mandated by our investors to seek business opportunities and projects for possible funding and debt capital financing.
Please note that our investors are from the Gulf region. They intend to invest in viable business ventures or projects that you are currently executing or intend to embark upon as a means of expanding your (their) global portfolio.
We are eager to have more discussions on this subject in any way you believe suitable.
Please contact me on my direct email: ahmed.abdulla@dejlaconsulting.com
Looking forward to working with you.
Yours faithfully,
Ahmed Abdulla
financial advisor
Dejla Consulting LLC
Коммерческий транспорт в лизинг с минимальным пакетом документов
купить в лизинг коммерческий автомобиль http://www.lizing-kommercheskogo-transporta1.ru .
Drones con luces danzantes creando magia en el cielo
drones iluminados show0-de-drones.com .
Нанесение надписей и изображений на футболки под заказ
футболки с надписями на заказ футболки с надписями на заказ .
prescription costs Administration guidelines here. Dosing guidelines here. best drug prices
Советы по подготовке к экзотическому отдыху.
Екзотични почивки http://www.ekzotichni-pochivki.com/ .
Bright and immersive drone light show tailored to your needs
drone show costs https://drone0-show.com .
cheap prescription drugs Drug pamphlet provided. Medication data provided. discount rx
Огромный выбор шин и дисков — от эконом до премиум
интернет магазин автомобильных шин shini-kupit-v-spb.ru .
prescription drug price check Pill overview available. Medication trends described. non prescription drugs
drug store online Patient medication leaflet. Pill effects explained. online prescription
best online international pharmacies Abuse effects detailed. Get pill facts. best online pharmacy stores
online pharmacies Read about medicines. Patient pill resource. internet pharmacy
safe online pharmacies in canada Medicine facts available. Drug pamphlet provided. online drugs
legitimate online pharmacies Access pill details. Medicine leaflet available. northwestpharmacy com
On this site presents CD/radio/clock combos made by trusted manufacturers.
You can find top-loading CD players with FM/AM reception and dual wake options.
Many models come with external audio inputs, device charging, and memory backup.
This collection covers value picks to elite choices.
alarm clock with usb music player
Each one include sleep timers, rest timers, and bright LED displays.
Buy now through eBay with fast shipping.
Discover the perfect clock-radio-CD setup for bedroom daily routines.
This website, you can discover lots of casino slots from leading developers.
Users can experience classic slots as well as feature-packed games with vivid animation and bonus rounds.
Even if you’re new or an experienced player, there’s something for everyone.
play casino
Each title are available round the clock and optimized for laptops and tablets alike.
No download is required, so you can start playing instantly.
The interface is intuitive, making it quick to find your favorite slot.
Sign up today, and discover the excitement of spinning reels!
safe online pharmacies in canada Medication details here. Latest drug developments. discount medicine
prescription costs Pill guide available. Medication data provided. pain meds on
pharmacies online Drug specifics here. Access medicine facts. drug store online
online pharmacies canada Pill guide available. Drug reactions explained. pharcharmy online no precipitation
canada drug pharmacy Medicine effects explained. Medication overview available. canadian pharmacies reviews
canadian drug prices Medication essentials explained. Medication reactions explained. canadian prescriptions
prescription drug costs Medication leaflet provided. Get medication details. price prescription
Thanks you.
https://hop.cx
us pharmacy no prior prescription Drug leaflet here. Medication facts provided. prescription online
prescription costs Medication guide here. Interactions explained here. canadian pharcharmy online
canadian pharmacy onli Comprehensive medication facts. Medicine facts available. prescription price checker
AI is Changing Search—Is Your Business Ready? AI and voice search now power over 50% of online traffic—but most websites aren’t optimized for it. If your site still relies on traditional SEO, you’re already losing visibility to AI-driven search results.
I help businesses stay searchable, visible, and competitive in this new landscape. Let’s do a free audit and see where you stand.
Email me at Admin@Marketer2025.com to book a time, or visit Marketer2025.com to learn more.
canada drugs online Drug guide here. Find pill information. canada drugs no prescription needed
pharmacy online mexico Latest pill trends. Prescribing guidelines here. legitimate canadian mail order pharmacies
Уважаемые клиенты! Мы рады сообщить, что теперь вы можете заказать авто напрямую в Японии, Китае и Кореи!
Обширный запас автомобилей от ключевых корейских, китайских, европейских, японских, и американских производителей.
Индивидуальный подбор автомобиля под ваши запросы.
Надёжная схема работы и установленная стоимость.
Кратчайшие сроки привоза.
Оформление на всех этапах: от поиска до постановки авто на учет.
Выдача документов включая чеки за оказанные агентские услуги.
Свяжитесь с нами
+79644340397
+79952187276
vttautonhk@gmail.com
rx online pharmacy Medication leaflet here. Comprehensive drug facts. www canadapharmacy com
international pharmacies that ship to the usa Medicine overview available. Drug trends described. prescription drug costs
mexican pharmacies shipping to usa Find medicine information. Drug facts provided. no prescription pharmacy
pharmacy without dr prescriptions Access medication facts. Complete drug overview. canadian drugs
planet drugs direct Medication facts provided. Side effects explained. canadian meds
top rated online canadian pharmacies Comprehensive pill overview. Pill facts available. prescriptions online
Drug leaflet here. https://cmqmeds.shop/# Find medicine information. canada pharmacy
Наличие медицинской страховки во время путешествия — это необходимая мера для обеспечения безопасности путешественника.
Страховка обеспечивает расходы на лечение в случае обострения болезни за границей.
Кроме того, полис может охватывать оплату на медицинскую эвакуацию.
merk-kirov.ru
Многие страны предусматривают наличие страховки для пересечения границы.
При отсутствии полиса обращение к врачу могут обойтись дорого.
Оформление полиса заранее
Pill info here. https://cmqmeds.shop/# Current medicine trends. canada pharmacies wit
Калькулятор досрочного погашения кредита учитывает все детали
досрочное погашение кредита калькулятор https://www.finanspro24.ru/ .
Не рискуйте: проверьте кредитный рейтинг перед подачей заявки в банк
узнать кредитный рейтинг бесплатно онлайн https://www.dengivperedservice.ru .
Снижение процентной ставки через рефинансирование кредита без потери баллов
как рефинансировать кредит как рефинансировать кредит .
Get medicine facts. https://cmqmeds.shop/# Medication impacts explained. best online canadian pharcharmy
This website offers you the chance to get in touch with experts for occasional dangerous projects.
Users can securely set up services for particular operations.
Each professional have expertise in handling intense tasks.
hitman-assassin-killer.com
This service offers secure connections between employers and specialists.
When you need fast support, this website is the perfect place.
Post your request and find a fit with an expert instantly!
Administration guidelines here. https://cmqmeds.shop/# Pill impacts described. online pharmacies canada
Latest medication news. https://isotretinoinfix.shop/# Latest pill developments. accutane fluconazole
Questo sito offre la selezione di operatori per incarichi rischiosi.
Chi cerca aiuto possono scegliere operatori competenti per operazioni isolate.
Tutti i lavoratori vengono verificati con cura.
sonsofanarchy-italia.com
Sul sito è possibile consultare disponibilità prima della selezione.
La professionalità è un nostro impegno.
Contattateci oggi stesso per ottenere aiuto specializzato!
accutane fraxel Complete pill overview. Medication details here. accutane cephalexin
Hi there, Things are rough for many businesses right now, which is why I’m offering a one-time, no-strings-attached outreach blast to 50,000 contact forms, completely free. This is the same method I use for my paying clients to generate leads fast, and I’m offering it free to help businesses during this downturn. If you’d like to claim one of the free spots, just visit https://free50ksubmissionsoffer.my, and I’ll handle everything for you. No cost, no commitment. Just an opportunity to help you get noticed in tough times.
Get medication facts. https://isotretinoinfix.shop/# Side effects explained. isotretinoin paypal
accutane sudafed Drug trends described. Medicine leaflet available. accutane mexican
Patient drug guide. https://isotretinoinfix.shop/# Medication essentials explained. accutane hollywood
На данной странице вы можете найти свежую ссылку 1xBet без блокировок.
Оперативно обновляем зеркала, чтобы предоставить стабильную работу к порталу.
Используя зеркало, вы сможете получать весь функционал без перебоев.
1xbet-official.live
Наш сайт поможет вам моментально перейти на новую ссылку 1 икс бет.
Нам важно, чтобы каждый пользователь смог работать без перебоев.
Следите за обновлениями, чтобы не терять доступ с 1xBet!
Premium Features:
? Ultra-Soft Skin: Mimics the touch and warmth of real human skin with medical-grade TPE material.
? Anatomically Precise Design: Proportional curves and lifelike details for unparalleled realism.
? Flexible Metal Frame: Adjustable joints for endless posing possibilities.
? Certified Safety: Non-toxic, odor-free materials tested and approved by CCIC.
? Full-Body Versatility: Designed for intimate exploration – vaginal, anal, oral, and beyond.
? Easy Maintenance: Effortless cleaning for lasting hygiene.
Exclusive AliExpress Offer: Secure your premium companion at a special price – stock is limited.
Order Now on AliExpress
Discreet & Secure: Shipped in plain packaging with total privacy guaranteed.
Crafted with Precision. Designed for Desire.
Elevate Your Experience – Order Today.
Наша платформа — официальный интернет-бутик Bottega Венета с доставлением по РФ.
На нашем сайте вы можете заказать брендовые изделия Боттега Венета официально.
Каждый заказ идут с официальной гарантией от производителя.
bottega veneta
Перевозка осуществляется быстро в любое место России.
Бутик онлайн предлагает выгодные условия покупки и гарантию возврата средств.
Положитесь на официальном сайте Bottega Veneta, чтобы получить безупречный сервис!
isotretinoin monitoring Medication resource here. Complete pill overview. accutane catapora
Patient medication resource. https://isotretinoinfix.shop/# Find medicine info. accutane maintenance
在这个网站上,您可以聘请专门从事单次的危险任务的人员。
我们整理了大量经验丰富的从业人员供您选择。
无论需要何种挑战,您都可以轻松找到合适的人选。
如何在网上下令谋杀
所有任务完成者均经过审核,保证您的利益。
服务中心注重安全,让您的个别项目更加无忧。
如果您需要详细资料,请与我们取得联系!
accutane cps Access medication details. Get info now. accutane plm
Кредитный рейтинг: как быстро исправить ошибки в кредитной истории
проверить кредитный рейтинг https://www.budgetmasterexpert.ru/ .
Comprehensive pill resource. https://ivermectinfix.shop/# Medication trends described. buy stromectol australia
buy Ivermectin no prescription Find medication details. Comprehensive medication resource. buy stromectol online
Find drug information. https://ivermectinfix.shop/# Read about medications. buy stromectol no prescription
buy stromectol no prescription Comprehensive drug resource. Formulation info listed. purchase Ivermectin
Here, you can explore trusted websites for CS:GO betting.
We list a wide range of wagering platforms dedicated to CS:GO.
Every website is tested for quality to guarantee safety.
cs 2 betting
Whether you’re a CS:GO enthusiast, you’ll effortlessly discover a platform that matches your preferences.
Our goal is to make it easy for you to find only the best CS:GO wagering platforms.
Explore our list at your convenience and elevate your CS:GO gambling experience!
Pill information available. https://ivermectinfix.shop/# Comprehensive drug facts. stromectol cheap
stromectol buy cheap Drug pamphlet provided. Dosing guidelines here. buy Ivermectin pills
Кредит без отказа: полный список банков с лучшими условиями
где можно взять кредит без отказа где можно взять кредит без отказа .
Get drug facts. https://ivermectinfix.shop/# Administration guidelines here. purchase stromectol
order stromectol Patient drug information. Pill information provided. buy stromectol
Pill guide available. https://ivermectinfix.shop/# Comprehensive medication guide. buy stromectol canada
Patient pill information. https://canadiantrustpharmacy.top/# Find pill facts. us pharmacy online
canada pharmacy no prescription Patient medicine info. Medicine facts here. medco best online pharmacy
Comprehensive medication overview. https://canadiantrustpharmacy.top/# Drug reactions explained. canadian pharmacy online reviews
best online pharmacy no prescription Patient pill facts. Latest pill updates. best online pharmacy oxycontin
Comprehensive drug facts. https://canadiantrustpharmacy.top/# Comprehensive drug overview. rite aid best online pharmacy
Оригинальные контрактные двигатели без пробега по РФ
контрактные двигатели купить https://www.kontraktnye-dvigateli1-minsk.ru/ .
Индивидуальные проекты каркасных домов с планировкой под клиента
строительство каркасных домов в санкт-петербурге https://karkasnye-doma-pod-kluch-spb.ru/ .
ritalin online pharmacy Pill information here. Get pill details. pharmacy tech school online
Medication details here. http://canada-pharmacyonline.top/# Latest medication developments. cheapest online pharmacy
Сервис по подбору бу запчастей по коду или названию детали
б у запчасти https://www.zapchasti-bu1-minsk.ru .
generic viagra online canada pharmacy Latest drug news. Access medicine facts. finasteride online pharmacy
Administration guidelines here. http://canada-pharmacyonline.top/# Patient pill information. generic viagra online canada pharmacy
non prescription pharmacy Recent medicine developments. Medication effects explained. tricare online pharmacy
Medicine guide here. https://erectiledysfunctionpills365.top/# Medicine leaflet here. ed medications
Premium Features:
– Ultra-Soft Skin: Mimics the touch and warmth of real human skin with medical-grade TPE material.
– Anatomically Precise Design: Proportional curves and lifelike details for unparalleled realism.
– Flexible Metal Frame: Adjustable joints for endless posing possibilities.
– Certified Safety: Non-toxic, odor-free materials tested and approved by CCIC.
– Full-Body Versatility: Designed for intimate exploration – vaginal, anal, oral, and beyond.
– Easy Maintenance: Effortless cleaning for lasting hygiene.
Exclusive AliExpress Offer: Secure your premium companion at a special price – stock is limited.
Order Now on AliExpress
Discreet & Secure: Shipped in plain packaging with total privacy guaranteed.
Crafted with Precision. Designed for Desire.
Elevate Your Experience – Order Today.
ed pills that really work Medication trends described. Find drug information. natural remedies for ed
Drug details provided. https://erectiledysfunctionpills365.top/# Find drug information. buying ed pills online
Контрактные запчасти с авторазборки с возможностью самовывоза
авторазборка в минске avtorazborka1-minsk.ru .
Яркие букеты с доставкой — быстро, красиво и без переплат
букет из гортензии цена https://www.cvety-s-dostavkoi.ru/rubric/gortenzii .
Полный контроль над строительством каркасного дома с фото и отчётами
дом каркасный под ключ https://karkasnye-doma-spb-pod-kluch.ru/ .
Реалистичные куклы бебета для развития чувств.
Евтини кукли бебета https://www.kukli-bebeta.com .
Эффективные методы расслабления с игрушками.
Антистрес фиджет играчки http://www.antistres-igrachki.com/ .
non prescription ed drugs Comprehensive pill overview. Comprehensive medicine overview. ed meds online without doctor prescription
Мягкая мебель утратила былй лоск? Воскрешение мягкой мебели на дому в СПб! Подарим вторую жизнь диванам, креслам и коврам их истинную красоту. Профессиональные средства и опытные мастера. Скидки первым клиентам! Детали ждут вас! Тапайте https://himchistka-divanov-spb24.ru – Чистка диванов СПб на дому
Желаете отправить букет без лишних хлопот?
Тогда вам стоит обратить внимание на интересную статью о https://mavashimisha.ru/novosti/34989-dostavka-cvetov-v-moskve-ot-azalianow-ocharovatelnye-bukety.html в Москве.
Это стильное решение для тех, кто ценит удобство.
Мы рекомендуем прочитать о том, как оформить заказ за пару минут.
Курьеры приезжают точно в срок, а цветы всегда свежие и красиво оформлены.
Выбирайте проверенное качество!
Medicine information provided. https://erectiledysfunctionpills365.top/# Detailed pill knowledge. ed pills otc
the best ed pills Medication impacts explained. Drug info here. ed pills
Коммерческий транспорт в лизинг с онлайн-заявкой и удалённой подачей документов
взять в лизинг грузовой автомобиль https://lizing-kommercheskogo-transporta1.ru/gruzovye-avtomobili .
Medicine facts here. https://erectiledysfunctionpills365.top/# Get medicine details. best ed treatment pills
best ed pills non prescription Medication guide here. Detailed pill knowledge. cheapest ed pills
Постройте каркасный дом своей мечты с профессиональной командой
каркасные дома строительство https://www.karkasnye-doma-msk-pod-kluch.ru/ .
Каркасные дома для ПМЖ с подведёнными коммуникациями и теплым полом
дома каркасные под ключ karkasnye-doma-msk-pod-kluch0.ru .
Read about pills. https://erectiledysfunctionpills365.top/# Medicine guide available. cheap erectile dysfunction pill
100% Free Website Traffic — No signup, no catch. Just submit and go. Open to the first 500 people only: https://freewebsitetrafficforever.top
best online pharmacy technician training Complete medicine overview. Recent medicine developments. online steroid pharmacy
Medicine brochure provided. http://bestonlinepharmacy.top/# Pill info here. best online pharmacy vicodin
Espectaculo de drones con luces sincronizadas para eventos inolvidables
compañía de espectáculos de luces con drones https://show0-de-drones.com .
online pharmacies Side effects listed. Access pill details. online rx pharmacy
Современные технологии печати на футболках для ярких решений
принт на футболке pechat-na-futbolkah1.ru .
Complete medicine overview. http://bestonlinepharmacy.top/# Medication effects explained. canadian pharmacy no rx
Калькулятор досрочного погашения: как быстро снизить долг
калькулятор кредита с досрочным погашением http://www.finanspro24.ru/ .
Шины и диски в розницу и оптом с доставкой
шины магазин shini-kupit-v-spb.ru .
french pharmacy online Get medicine info. Get info now. tramadol best online pharmacy
Как проверить кредитный рейтинг и использовать его для улучшения условий займа
кредитный рейтинг бесплатно dengivperedservice.ru .
антенна TV Flat HD http://tv-antenka.ru .
Prescribing guidelines here. http://bestonlinepharmacy.top/# Patient medicine info. best online pharmacy technician training
pharmacy technician programs online Get pill facts. Patient drug leaflet. mexican pharmacies online
В данном ресурсе вы найдёте подробную информацию о реферальной системе: 1win партнерская программа.
Доступны все нюансы партнёрства, требования к участникам и возможные поощрения.
Каждый раздел детально описан, что помогает быстро усвоить в нюансах функционирования.
Плюс ко всему, имеются ответы на частые вопросы и подсказки для начинающих.
Материалы поддерживаются в актуальном состоянии, поэтому вы доверять в актуальности предоставленных данных.
Данный сайт окажет поддержку в изучении партнёрской программы 1Win.
Drug reactions explained. http://bestonlinepharmacy.top/# Get information instantly. latisse best online pharmacy
legit best online pharmacy Latest medicine developments. Medicine information provided. canadian best online pharmacy
Interactions explained here. http://bestonlinepharmacy.top/# Medicine effects explained. reputable online pharmacies
best online pharmacy paypal Read about medications. Get drug facts. best online pharmacy oxycodone 30mg
Pill trends described. http://bestonlinepharmacy.top/# Latest medication developments. online steroid pharmacy
online discount pharmacy Get pill facts. Medication data provided. best online pharmacy vicodin
Pill details provided. http://bestonlinepharmacy.top/# Active ingredients listed. legit best online pharmacy
online pharmacy tech programs Comprehensive medicine overview. Comprehensive medicine guide. trusted online pharmacy reviews
Latest medicine news. https://pampharma.top/# Read about drugs. online pharmacies
cvs pharmacy online application Find drug details. Pill overview available. mexican online pharmacy reviews
Recent drug developments. https://pampharma.top/# Get details now. cheap online pharmacy
mexica online pharmacy Short-term impacts described. Comprehensive medicine facts. online pharmacies mexica
Эскорт услуги в Санкт-Петербурге — Escort Piter https://escort-piter.com/
Drug leaflet available. https://pampharma.top/# Drug facts here. cheap online pharmacy
pharmacy online Medication overview available. Comprehensive medicine overview. pharmacy technician schools online
Medication leaflet here. https://pampharma.top/# Drug overview available. online mexican pharmacy
Почему каркасный дом — выгодный выбор для постоянного проживания
каркасный дом в спб https://www.karkasnye-doma-pod-kluch-spb.ru/ .
Заказывайте каркасный дом с фундаментом и кровлей по выгодной цене
каркасные дома в спб http://www.karkasnye-doma-spb-pod-kluch.ru/ .
buy slimex cheap Current medicine trends. Pill guide available. buy slimex cheap
Contraindications explained here. http://slimex-sibutramine.top/# Access medication facts. slimex online
Our service offers you the chance to get in touch with experts for short-term risky missions.
Clients may easily request help for particular needs.
All workers have expertise in handling sensitive operations.
hitman-assassin-killer.com
This service guarantees discreet connections between clients and workers.
Whether you need immediate help, this platform is the right choice.
Submit a task and get matched with an expert today!
Il nostro servizio rende possibile l’ingaggio di professionisti per incarichi rischiosi.
Chi cerca aiuto possono ingaggiare esperti affidabili per lavori una tantum.
Ogni candidato vengono verificati secondo criteri di sicurezza.
assumi assassino
Utilizzando il servizio è possibile consultare disponibilità prima di assumere.
La professionalità continua a essere un nostro valore fondamentale.
Esplorate le offerte oggi stesso per trovare il supporto necessario!
buy slimex with no prescription Drug specifics here. Drug information available. buy slimex uk
Latest pill news. http://slimex-sibutramine.top/# Comprehensive drug resource. buy sibutramine pills
sibutramine Medication effects explained. Drug leaflet available. order sibutramine
Drug leaflet here. http://slimex-sibutramine.top/# Pill guide available. slimex online
Выразите себя с помощью печати на футболке
заказ футболки со своим принтом https://www.pechat-na-futbolkah1.ru/ .
slimex online Drug trends described. Medicine leaflet here. slimex cheap
Overdose effects detailed. https://stromectol3us.top/# Side effects explained. ivermectin uk
https://www.adobe.com/
ivermectin 0.2mg Get pill details. Drug resource available. stromectol tablets for humans for sale
Medicine effects explained. https://stromectol3us.top/# Latest drug developments. ivermectin 1mg
ivermectin 3mg pill Side effects listed. Medicine details here. ivermectin lice oral
Кредитный рейтинг: что важно знать для успешного финансового планирования
узнать кредитный рейтинг https://www.budgetmasterexpert.ru/ .
Recent drug developments. https://stromectol3us.top/# Comprehensive medication overview. ivermectin 4000
buy ivermectin for humans uk Latest medication news. Get info now. stromectol tablets 3 mg
Complete medication overview. https://stromectol3us.top/# Medicine impacts described. stromectol ireland
ivermectin 9 mg Medication leaflet here. Patient drug information. ivermectin uk
Looking for experienced contractors ready to handle temporary hazardous tasks.
Require someone for a high-risk job? Find trusted individuals via this site to manage time-sensitive risky operations.
order a kill
This website matches employers with licensed workers willing to accept hazardous one-off positions.
Recruit pre-screened freelancers to perform risky tasks efficiently. Ideal when you need urgent scenarios demanding safety-focused skills.
Generic names listed. https://stromectol3us.top/# Medication pamphlet available. buy stromectol pills
Hello there! mexican pharmacy great web site.
Pill guide here. https://mexicanonlinepharmacies.top/# Drug facts here. pharm online
mexican pharm online Medication essentials explained. Access medicine facts. mexican pharmacy
mexican pharm online Medication guide available. Access drug facts. mexican pharm
# Harvard University: A Legacy of Excellence and Innovation
## A Brief History of Harvard University
Founded in 1636, **Harvard University** is the oldest and one of
the most prestigious higher education institutions in the United States.
Located in Cambridge, Massachusetts, Harvard has built
a global reputation for academic excellence,
groundbreaking research, and influential alumni.
From its humble beginnings as a small college established to educate clergy, it has evolved
into a world-leading university that shapes the future
across various disciplines.
## Harvard’s Impact on Education and Research
Harvard is synonymous with **innovation and intellectual leadership**.
The university boasts:
– **12 degree-granting schools**, including the renowned **Harvard Business
School**, **Harvard Law School**, and **Harvard Medical School**.
– **A faculty of world-class scholars**, many of whom are Nobel laureates,
Pulitzer Prize winners, and pioneers in their fields.
– **Cutting-edge research**, with Harvard leading initiatives in artificial intelligence, public
health, climate change, and more.
Harvard’s contribution to research is immense, with billions of dollars allocated to scientific discoveries and technological advancements each year.
## Notable Alumni: The Leaders of Today and Tomorrow
Harvard has produced some of the **most influential figures** in history,
spanning politics, business, entertainment, and science.
Among them are:
– **Barack Obama & John F. Kennedy** – Former U.S. Presidents
– **Mark Zuckerberg & Bill Gates** – Tech visionaries
(though Gates did not graduate)
– **Natalie Portman & Matt Damon** – Hollywood icons
– **Malala Yousafzai** – Nobel Prize-winning activist
The university continues to cultivate future leaders who shape industries and drive global progress.
## Harvard’s Stunning Campus and Iconic Library
Harvard’s campus is a blend of **historical charm and modern innovation**.
With over **200 buildings**, it features:
– The **Harvard Yard**, home to the iconic **John Harvard Statue** (and the famous “three
lies” legend).
– The **Widener Library**, one of the largest university libraries in the world, housing **over 20 million volumes**.
– State-of-the-art research centers, museums, and performing arts venues.
## Harvard Traditions and Student Life
Harvard offers a **rich student experience**, blending
academics with vibrant traditions, including:
– **Housing system:** Students live in one of 12 residential houses,
fostering a strong sense of community.
– **Annual Primal Scream:** A unique tradition where students de-stress by running
through Harvard Yard before finals!
– **The Harvard-Yale Game:** A historic football rivalry
that unites alumni and students.
With over **450 student organizations**, Harvard students engage in a diverse range of extracurricular activities,
from entrepreneurship to performing arts.
## Harvard’s Global Influence
Beyond academics, Harvard drives change in **global policy, economics, and technology**.
The university’s research impacts healthcare, sustainability,
and artificial intelligence, with partnerships across industries worldwide.
**Harvard’s endowment**, the largest of any university, allows it
to fund scholarships, research, and public initiatives, ensuring a legacy of impact for generations.
## Conclusion
Harvard University is more than just a school—it’s a **symbol of
excellence, innovation, and leadership**. Its **centuries-old traditions, groundbreaking discoveries, and transformative education** make it one
of the most influential institutions in the world.
Whether through its distinguished alumni, pioneering research, or
vibrant student life, Harvard continues to shape the future in profound ways.
Would you like to join the ranks of Harvard’s legendary scholars?
The journey starts with a dream—and an application!
https://www.harvard.edu/
Order a light drone show that adds elegance and energy to your night
drone show price drone show price .
Get drug info. https://mexicanonlinepharmacies.top/# Patient medicine guide. mexican pharm
Produccion completa de shows con drones y efectos especiales
espectaculo drones show0-de-drones.com .
mexican pharmacy Detailed pill knowledge. Drug leaflet available. mexican pharm
Europese apotheek
Pregamid kopen zonder recept! => https://bit.ly/elyrica
— Lage prijzen voor geneesmiddelen van hoge kwaliteit
— Snelle levering en volledige vertrouwelijkheid
— Bonuspillen en grote kortingen bij elke bestelling
— Uw volledige tevredenheid gegarandeerd of uw geld terug
.
.
.
.
.
Pregamid 300mg prijs Pregamid 300mg lage prijs
Pregamid 300mg kopen
goedkoop Pregamid 300 mg Waar te koop Pregamid 300mg
Pregamid 300 mg lage prijs
Pregamid 300mg kopen koop goedkoop Pregamid 300mg
Pregamid 300mg kopen zonder recept
goedkoop Pregamid 300mg Waar te bestellen Pregamid 300 mg
Waar te koop Pregamid 300 mg Pregamid 300mg kopen
Waar kan ik Pregamid 300mg kopen Pregamid 300 mg prijs
Pregamid 300 mg lage prijs Pregamid 300 mg zonder recept
Pregamid 300mg lage prijs Pregamid 300mg lage prijs
Pregamid 300 mg kopen
Waar te bestellen Pregamid 300mg
Drug impacts explained. https://mexicanonlinepharmacies.top/# Latest pill trends. mexican pharm online
On this platform, you can discover a great variety of casino slots from top providers.
Players can experience classic slots as well as feature-packed games with high-quality visuals and exciting features.
Whether you’re a beginner or a seasoned gamer, there’s always a slot to match your mood.
casino games
Each title are ready to play round the clock and compatible with laptops and mobile devices alike.
No download is required, so you can get started without hassle.
The interface is user-friendly, making it convenient to explore new games.
Sign up today, and dive into the excitement of spinning reels!
Latest pill developments. https://www.google.cf/url?sa=t&url=https%3A%2F%2Fmexicanonlinepharmacies.top Medicine impacts described. mexican pharmacy
mexican pharm online Drug pamphlet provided. Find medicine information. pharm online
Professional-looking rubber stamps online for free
stamp creator online stamp creator online .
Pill leaflet here. https://abrazpharmacy.top Pill facts available. legit online pharmacy
WoM nhP zKUsnJhk LsVhccjL qzgrsP DjZ
People consider ending their life due to many factors, frequently arising from intense psychological suffering.
Feelings of hopelessness may consume someone’s will to live. In many cases, lack of support is a major factor to this choice.
Conditions like depression or anxiety distort thinking, making it hard for individuals to see alternatives beyond their current state.
how to kill yourself
External pressures might further drive an individual closer to the edge.
Limited availability of resources may leave them feeling trapped. Understand getting help makes all the difference.
viagra online mexican pharmacy Find medication info. Medicine effects explained. best online pharmacy
Read about drugs. https://abrazpharmacy.top Detailed drug knowledge. online pharmacy mexica
adderall online pharmacy Pill effects explained. Medicine effects explained. mexican pharmacy
Девушки эскортницы благовидные, умные и свободные
Среди огромного количества профессий современного мира одна из самых загадочных, спорных и окутанных большим множеством мифов это профессия эскортницы. Девушки, работающие в эскорте недорого шлюхи Санкт-Петербург эксклюзивные всегда вызывали живой энтузиазм и восхищение находящийся вокруг. Их красота, стиль, умение себя преподнести и держаться между делают их реальными музами для многих.
Что такое эскорт
Эскорт это сопровождение клиента на светские мероприятия, деловые встречи, путешествия или вечеринки. Вопреки распространённым заблуждениям, эскорт не всегда связан с интимом. Чаще всего девушки-эскортницы это умственные и компанейские люди, способные поддержать беседу на любую тему, а ещё стать безупречным спутником для успешного мужчины.
Comprehensive pill guide. https://abrazpharmacy.top Comprehensive medicine guide. online pharmacy school
Выгодное рефинансирование кредита с прозрачными условиями и без скрытых комиссий
сделать рефинансирование кредита под меньший процент http://www.kapitalinfo-team.ru .
Секреты выбора императорского фарфора: что стоит учесть при покупке
фарфоровый завод https://www.imperatorskij-farfor.website.yandexcloud.net/ .
Всё для идеальной дегустации: бокалы для вина в наличии и под заказ
бокал под вино https://bokaly-dlya-vina.website.yandexcloud.net .
online pharmacy mexica Find drug information. Get pill facts. mexican pharmacy
Find pill info. https://canadianpharmeasy.shop/# Pill facts available. canadian pharm online
canadian pharm Drug trends described. Medication trends described. online pharmacy school
Pill impacts explained. https://canadianpharmeasy.shop/# Comprehensive medicine facts. adderall online pharmacy
DIY stamp design is simple with a rubber stamp maker online
stamp maker online free make1-stamps-online.com .
[url=https://canadianpharmeasy.shop/#]cialis online pharmacy[/url] Comprehensive drug resource. Latest medication news. online pharmacy no prescription
Medicine resource available. https://images.google.sm/url?sa=t&url=https%3A%2F%2Fcanadianpharmeasy.shop Pill facts available. viagra online canadian pharmacy
online pharmacy viagra Brand names listed. Get pill details. mexican pharm online
Get medication facts. http://www.bssystems.org/url?q=https%3A%2F%2Fonlinepharmeasy.shop Find pill info. mexican online pharmacy
Free rubber stamp design software online – no installation needed
create stamp online free https://mystampready-constructor1.com .
[url=https://onlinepharmeasy.shop/#]pharm online[/url] Latest pill updates. Medicine impacts explained. walgreens online pharmacy